C# Date/Time Property
using System
DateTime.UtcNow;
Internationalization (I18n) Property Overview
The DateTime.UtcNow property is the
DateTime object encapsulating
the current, local date and time on this computer, expressed as the Coordinated Universal Time (UTC).
See Microsoft's
MSDN online documentation for more information.
I18n Issues
Because DateTime
objects are culture-sensitive, developers retrieving
this property need to ensure that the
CultureInfo has been set
properly in the current thread, which would in turn ensure that the
DateTime.UtcNow object was instantiated to use the correct
set of culture-specific rules.
It is also important to check code that uses the DateTime.UtcNow
property to make sure that pieces of the current DateTime aren't being pasted
together in a locale-specific way, as in the case of the incorrect usage
example below.
Usage
The following example demonstrates the wrong way to use the
DateTime.UtcNow property. This code example pieces together
a display date in a fashion that is specific to U.S. English. It will
be incorrect for even other English-speaking locales, such as the United
Kingdom.
string theMonth = DateTime.UtcNow.Month.ToString();
string theDay = DateTime.UtcNow.Day.ToString();
string theYear = DateTime.UtcNow.Year.ToString();
string theTime = DateTime.UtcNow.Hour.ToString() + ":" +
DateTime.UtcNow.Minute.ToString() + ":" +
DateTime.UtcNow.Second.ToString();
string displayTime = "Timestamp: " +
theTime + " " + theMonth + "/" + theDay + "/" + theYear;
The correct way to format a long date string such as the one
attempted above, would be to call the ToString method
on DateTime.UtcNow , passing in the D format
string, which specifies a long date value. This way, if the correct
culture has been set in the current thread, the date formatting rules
of the user's culture will be used to generate the correct long-date
string. Even better, it's done in a single line of code instead of
eight:
string displayTime = DateTime.UtcNow.ToString("D");
General C# date/time formatting information
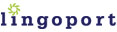
|