C# Type Conversion
using System
public string ToString();
public string ToString(IFormatProvider provider);
public string ToString(string format);
public string ToString(string format, IFormatProvider provider);
Internationalization (I18n) Method Overview
Frequently you will want to display to your users a string
version of a date, number or other data type within your code. In these cases you
will call the ToString() method on that variable to
generate a display string.
For more information see Microsoft's MSDN online documentation
here and here.
I18n Issues
Most base classes within C# override this method,
allowing the user to specify a format string along with a
CultureInfo
object (which is a subclass of IFormatProvider ) to generate a string formatted according to certain
culture-specific rules.
If no CultureInfo is provided, ToString() will draw from
the CultureInfo object that has been set in the current thread.
Globalyzer detects these calls to prompt developers
to double check that they are using the correct CultureInfo to perform
this formatting functionality.
Usage
The following code example uses the CultureInfo class to specify the culture
that the ToString() method and format string will use. This code creates a new
instance of the CultureInfo class called MyCulture and initializes
it to the French culture using the string fr-FR. This object is passed to the
ToString()
method with the C string format specifier to produce a French monetary value.
int MyInt = 100;
CultureInfo MyCulture =
new CultureInfo("fr-FR");
String MyString =
MyInt.ToString("C", MyCulture);
Console.WriteLine(MyString);
The preceding code displays 100,00 € when displayed in a Windows Forms form.
Note that the console environment does not support all Unicode characters and
displays 100,00 ? instead.
The following table lists the format characters available to users who call
the ToString() method:
Format Character |
Description
|
C, c |
Currency |
D, d |
Decimal |
E, e |
Scientific (exponential) |
F, f |
Fixed point |
G, g |
General |
N, n |
Number |
P, p |
Percentage |
R, r |
Round trip to and from strings |
X, x |
Hexadecimal |
C# Type Conversions
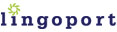
|